- Phone Interview Cheat Sheet
- Technical Interview Cheat Sheet 2020
- Technical Interview Cheat Sheet Reddit
It helps me to feel calm and confident before an interview. And it is curated with the intent of covering every single detail so I know for sure that I have done my absolute best to get prepared. You can expect to find in here logistic tips, bathroom schedules, makeup tutorials, and technical cheat sheet. Bitwise operators Operation. X y Returns x with the bits shifted to the right by y places. This is the same as //'ing x by 2.y. X & y Does a “bitwise and”. Each bit of the output is 1 if the corresponding bit of x AND of y is 1.
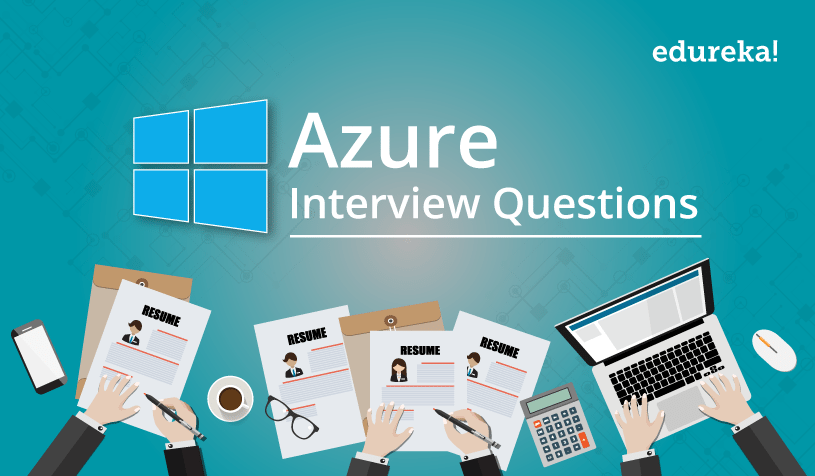
This blog post is a coding and system design interview cookbook/cheatsheet compiled by me with code pieces written by me as well. There is no particular structure nor do I pretend this to be a comprehensive guide. Also there are no explanations as the purpose is just to list important things. Moreover, this blog post is work-in-progress as I’m planning to add more things to it.
As always, opinions are mine.
Binary Search
Breadth First Search
Depth First Search
Just replace the queue in BFS with stack and you are done:
Backtracking
Generally a recursive algorithm attempting candidates and either accepting them or rejecting (“backtracking”) based on some condition. Below is an example of permutations generation using backtracking:
Dynamic Programming
Technique to determine result using previous results. You should be able to reason about your solution recursively and be able to deduce dp[i] using dp[i-1]. Generally you would have something like this:
Dynamic Programming: Knapsack
Union Find
When you need to figure out if something belongs to the same set you can use the following:
Binary Tree Traversals
- Inorder: left-root-right
- Postorder: left-rigth-root
- Preorder: root-left-right
- Plus each one of them could have reverse for right and left
Below are recursive and iterative versions of inorder traversal
Segment Tree
Djikstra
Simplest way to remember Djikstra is to imagine it as BFS over PriorityQueue instead of normal Queue, where values in queue are best distances to nodes pending processing.
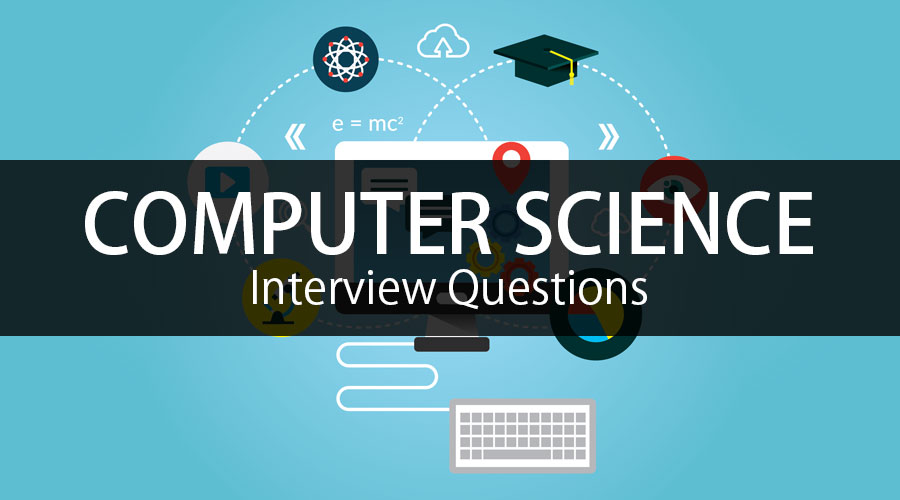
Multi-threading: Semaphore
Multi-threading: Runnable & synchronized
Strings: KMP
Hopefully no one asks you this one. It would be too much code here, but the idea is not that difficult: build LPS (example “AAABAAA” -> [0,1,2,0,1,2,3]); use LPS to skip when comparing as if you were doing it in brute-force.
Strings: Rolling Hash (Rabin-Karp)
Let’s imagine we have window of length k and string s, then the hash for the first window will be:
Adobe premiere pro and after effects free download. H(k) = s[0]*P^(k-1) + s[1]*P^(k-2) + … + s[k]*P^0 Affinity ipad.
Moving the window by one, would be O(1) operation:
H(k+1) = (H(k) – s[0]*P^(k-1)) * P + s[k+1]*P^0
Here is some sample code:
Unit Testing
JUnit User guide: https://junit.org/junit5/docs/current/user-guide/
Data Structures
Many interview problems can be solved by using right data structure.
- hashmap
- stack
- queue
- priority queue
- trees
- linked list
- …
- Actually, better to go here: https://www.geeksforgeeks.org/data-structures/
System Design Topics
- requirements gathering
- capacity planning
- high level design
- components design
- database design
- API design
- queue
- map reduce
- long poll and push models
- load balancing
- partitioning
- replication
- consistency
- caching
- distributed caching
- networking
Some System Design Problems
- TinyURL
- Coming up with good encoding or better pre-building keys
- Handling collisions (if encoding or if different users have to have different key)
- Use distributed caching, CDN, LB
- Consider API throttling, eviction (maybe not), capacity
- HTTP 302
- Pastebin
- Separate data from metadata and store separately
- Otherwise similar to TinyURL
- Instagram
- Data partitioning is important here
- Pull vs push (via long polling) applied based on number of following
- Separate servers for viewing and posting for stability
- Generating feed of images requires sorting, consider timestamp for photoId
- Dropbox
- Client and Server
- Splitting files into chunks
- Saving metadata about chunks
- Local metadata database for the client
- Queues for load handling
- Data deduplication (user has same file in 100 places)
- Facebook Messenger
- poll vs push model with long polling
- push notifications
- Dedicate chat servers per user
- “seeing into the future” problem solving with seq number
- active status
- Twitter
- prebuild feed
- handle celebrities differently
- caching
- monitoring
- Youtube or Netflix
- Splitting files into chunks (time and quality) via MapReduce
- Distribution of content
- Thumbnail generator & distribution
- Store metadata separately (likes, views, comments, etc)
- CDN
- Typeahead Suggestion
- Trie
- For frequencies store them in nodes with suggestions
- Propagate suggestions independently from queries (mapreduce)
- Client implementation should delay request allowing for typing
- Client having local history
- API Rate Limiter
- DDoS
- Client identifier
- Sliding window with counters
- HTTP 429
- Web Crawler
- BFS
- Facebook’s Newsfeed
- Different feed components stored separately but joined
- Yelp
- Quad-Tree
- Uber
- Dynamic Quad-Tree
- HashMap
- Ticketmaster
- Relational DB design
- Concurrency handling
Resources

Well, internet is just full of all kinds of resources and blog posts on how to prepare for the technical interview plus there are numerous books and websites available. LeetCode is probably the most known of practicing websites. “Cracking The Coding Interview” is probably the most known book. I can also recommend “Guide to Competitive Programming”. As of system design, preparation resources are not so readily available. One good resource is “Grokking System Design Interview”, but it would be more valuable to practice designing large distributed systems. I also found the book “Designing Data-Intensive Applications” to be extremely helpful.
Asking for help
What are other “must know” algorithms and important system design topics to be listed in this post?
This is a straight-to-the-point, distilled list of technical interview Do's and Don'ts for interviewers, mainly for algorithmic interviews. Some of these may apply to only phone screens or whiteboard interviews, but most will apply to both. I revise this list before each of my interviews as an interviewer to remind myself of them and eventually internalized all of them to the point I do not have to rely on it anymore.
If you are a candidate you probably do not need to know this section well. You may read this to get a better sense of what an interviewer is supposed to do during an interview.
Legend#
- ✅ = Do
- ❌ = Don't
- ⚠️ = Situational
Before interview#
Things | |
---|---|
✅ | Make sure your surroundings are well-lit. |
✅ | Find a quiet environment with good Internet connection. |
✅ | Ensure webcam and audio are working. Test that your VC app is working well. |
✅ | Prepare two to three questions and be familiar with the different approaches for solving the questions. |
✅ | Familiarize yourself with the coding environment (CoderPad/CodePen). Set up the coding shortcuts, turn on autocompletion, tab spacing, etc. |
Introduction#
Things | |
---|---|
✅ | Check if candidate wants to use the restroom or take a break. |
✅ | Give an overview of the interview format (introduction, duration, programming languages available, 5 min at the end for Q&A). |
✅ | Do a self-introduction and get the candidate to introduce themselves. |
✅ | Explain to candidate that they do not have to finish all questions and you might interrupt them abruptly. |
❌ | Allow the candidate to spend too long introducing themselves. |
Upon delivering the question#
Things | |
---|---|
✅ | Ask if the candidate has seen the question before. |
✅ | Give a small example for the question and the desired output. |
✅ | Get the candidate to talk through the solution first before diving into coding. |
✅ | Provide hints where appropriate. |
✅ | If candidate is still stuck after providing hints, provide the solution and move to coding so that you can get coding signals. |
During coding#
Things | |
---|---|
✅ | If whiteboard interview, stand alongside candidate but also giving them space, instead of being distant, e.g seated down. |
✅ | Take note of all the positive and negative signals. |
❌ | Check the time in an overly-obvious manner. |
Phone Interview Cheat Sheet
After coding#
Technical Interview Cheat Sheet 2020
Things | |
---|---|
✅ | Ask for candidate to provide test cases and run through the code with them. |
✅ | Point out edge cases candidate missed and ask the candidate to address them. |
✅ | Take note of the duration TC spent on each question to include in the feedback. |
✅ | Ask for time complexity and space analysis. |
✅ | Preserve the code somewhere - take a picture or copy the code out. |
✅ | Stop the candidate when there is 5 minutes left. e.g. ('I'll stop you here and let's go to the next section') |
Wrap up#
Things | |
---|---|
✅ | Allow candidate to ask questions and answer them to the best of your ability. |
✅ | Thank the candidate and wish them all the best. |
Post interview#
Technical Interview Cheat Sheet Reddit
Things | |
---|---|
✅ | Write feedback as soon as possible to not forget details. |
